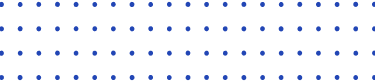
QuickFix/C++ Documentation
with Python and Ruby
Installation
Dependencies
Building
Windows
Open quickfix_vs12.sln, quickfix_vs14.sln, or quickfix_vs15.sln
Batch build all projects. lib\quickfix.lib and lib\debug\quickfix.lib can be linked into your application. Header files are copied to the include directory.
Compile time options are controlled from the config_windows.h file in the src directory. The following options are supported:
#define HAVE_STLPORT 1 |
Compile with stlport instead of standard visual c++ STL implementation. |
#define HAVE_ODBC 1 |
Compiles ODBC support into QuickFIX. |
#define HAVE_MYSQL 1 |
Compiles MySQL support into QuickFIX. If you enable this option, the mysql include and library directories must be in the Visual Studio search paths. |
#define HAVE_POSTGRESQL 1 |
Compiles PostgreSQL support into QuickFIX. If you enable this option, the postgres include and library directories must be in the Visual Studio search paths. |
Linux / Solaris / FreeBSD / Mac OS X
./configure && ./make sudo make install
You can also run configure with the --prefix=<base directory> option to install to a custom location.
./configure options:
--with-python2 |
Build the Python2 API |
--with-python3 |
Build the Python3 API |
--with-ruby |
Build the Ruby API |
--with-mysql |
Compile in mysql support |
--with-postgresql |
Compile in postgresql support |
--with-stlport=<base directory> |
Compile with stlport instead of standard gcc STL implementation |
When using the SUNPro compiler:
CC=<full path to SUNPro CC binary>
CFLAGS=-g -xs
CXX=<full path to SUNPro CC binary>
CXXFLAGS=-g -xs
LD=<full path to SUNPro CC binary>
LDFLAGS=-g -xs -lCstd
AR=<full path to SUNPro CC binary>
AR_FLAGS=-g -xs -xar -o
Generating Databases
MySQL
You must have MySQL installed with the deamon running.
Run the create script in src/sql/mysql. You must pass in a mysql user with permissions authorized to create a new database.
./create.sh mysql
create.bat mysql
MSSQL
You must have MySQL installed with the deamon running.
Run the create script in src/sql/mssql. You must pass in a mssql user with permissions authorized to create a new database.
create.bat sa
PostgreSQL
You must have PostgreSQL installed with the deamon running.
Run the create script in src/sql/postgresql. You must pass in a postgresql user with permissions authorized to create a new database.
./create.sh postgres
create.bat postgres
Testing
The development of QuickFIX has been driven by a suite of functional acceptance tests and unit tests.
Windows
Unit Tests
From the test directory:
runut release [port]
The port is used to test socket functionality. If you built QuickFIX with one of its supporting databases, you will need to update cfg/ut.cfg to reflect your database settings.
Acceptance Tests
From the test directory:
runat release [port]
runat_threaded release [port]
The port is used to listen for connections on a socket server.
Linux / Solaris / FreeBSD / Mac OS X
Unit Tests
From the test directory:
./runut.sh [port]
The port is used to test socket functionality. If you built QuickFIX with one of its supporting databases, you will need to update cfg/ut.cfg to reflect your database settings.
Acceptance Tests
From the test directory:
./runat.sh [port]
./runat_threaded.sh [port]
The port is used to listen for connections on a socket server.
Getting Started
Setting Up Your Project
Windows
Open project | properties in Microsoft Visual Studio.
- C/C++ | Code Generation | Enable C++ Exceptions, should be Yes.
- C/C++ | Code Generation | Runtime Library, should be set to Multithreaded DLL or Debug Multithreaded DLL.
- C/C++ | General | Additional Include Directories add the root quickfix directory.
- Linker | Input | Additional Dependencies, must contain quickfix.lib and ws2_32.lib.
- Linker | General | Additional Library Directories, add the quickfix/lib directory.
Linux/Solaris
- Exceptions must be enabled with -fexceptions
- The -finline-functions optimization is recommended.
- Quickfix library must be linked in with -lquickfix
- Quickfix makes use of pthreads and libxml. These must be linked in with -lpthread, -lxml2 and -lz
- On Solaris you must link with -lnsl and -lsocket
- GNU ld is a one-pass linker. Put more generally useful libraries (libsocket) last on the command line.
Creating Your Application
Creating a FIX application is as easy as implementing the QuickFIX Application interface. The C++ interface is defined in the following code segment:
C++
namespace FIX { class Application { public: virtual ~Application() {}; virtual void onCreate( const SessionID& ) = 0; virtual void onLogon( const SessionID& ) = 0; virtual void onLogout( const SessionID& ) = 0; virtual void toAdmin( Message&, const SessionID& ) = 0; virtual void toApp( Message&, const SessionID& ) throw( DoNotSend ) = 0; virtual void fromAdmin( const Message&, const SessionID& ) throw( FieldNotFound, IncorrectDataFormat, IncorrectTagValue, RejectLogon ) = 0; virtual void fromApp( const Message&, const SessionID& ) throw( FieldNotFound, IncorrectDataFormat, IncorrectTagValue, UnsupportedMessageType ) = 0; }; }
Python
class Application(_object): def onCreate(self, sessionID): return def onLogon(self, sessionID): return def onLogout(self, sessionID): return def toAdmin(self, message, sessionID): return def toApp(self, message, sessionID): return def fromAdmin(self, message, sessionID): return def fromApp(self, message, sessionID): return
Ruby
class Application < Quickfix::Application def onCreate(sessionID) end def onLogon(sessionID) end def onLogout(sessionID) end def toAdmin(sessionID, message) end def toApp(sessionID, message) end def fromAdmin(sessionID, message) end def fromApp(sessionID, message) end end
These methods act as callbacks from your FIX
sessions.
onCreate is called when quickfix creates a new
session. A session comes into and remains in existence for
the life of the application. Sessions exist whether or not a
counter party is connected to it. As soon as a session is
created, you can begin sending messages to it. If no one is
logged on, the messages will be sent at the time a connection
is established with the counterparty.
onLogon notifies you when a valid logon has
been established with a counter party. This is called when a
connection has been established and the FIX logon process has
completed with both parties exchanging valid logon
messages.
onLogout notifies you when an FIX session is no
longer online. This could happen during a normal logout
exchange or because of a forced termination or a loss of
network connection.
toAdmin provides you with a peek at the
administrative messages that are being sent from your FIX
engine to the counter party. This is normally not useful for
an application however it is provided for any logging you may
wish to do. Notice that the FIX::Message is not const. This
allows you to add fields to an adminstrative message before
it is sent out.
toApp is a callback for application messages
that are being sent to a counterparty. If you throw a
DoNotSend exception in this function, the application
will not send the message. This is mostly useful if the
application has been asked to resend a message such as an
order that is no longer relevant for the current market.
Messages that are being resent are marked with the
PossDupFlag in the header set to true; If a
DoNotSend exception is thrown and the flag is set to
true, a sequence reset will be sent in place of the message.
If it is set to false, the message will simply not be sent.
Notice that the FIX::Message is not const. This allows you to
add fields to an application message before it is sent
out.
fromAdmin notifies you when an administrative
message is sent from a counterparty to your FIX engine. This
can be usefull for doing extra validation on logon messages
like validating passwords. Throwing a RejectLogon
exception will disconnect the counterparty.
fromApp receives application level request. If
your application is a sell-side OMS, this is where you will
get your new order requests. If you were a buy side, you
would get your execution reports here. If a
FieldNotFound exception is thrown, the counterparty
will receive a reject indicating a conditionally required
field is missing. The Message class will throw this exception
when trying to retrieve a missing field, so you will rarely
need the throw this explicitly. You can also throw an
UnsupportedMessageType exception. This will result in
the counterparty getting a reject informing them your
application cannot process those types of messages. An
IncorrectTagValue can also be thrown if a field
contains a value you do not support.
The sample code below shows how you might start up a FIX
acceptor which listens on a socket. If you wanted an initiator,
you would replace the acceptor in this code fragment with a
SocketInitiator.
C++
#include "quickfix/FileStore.h" #include "quickfix/FileLog.h" #include "quickfix/SocketAcceptor.h" #include "quickfix/Session.h" #include "quickfix/SessionSettings.h" #include "quickfix/Application.h" int main( int argc, char** argv ) { try { if(argc < 2) return 1; std::string fileName = argv[1]; FIX::SessionSettings settings(fileName); MyApplication application; FIX::FileStoreFactory storeFactory(settings); FIX::FileLogFactory logFactory(settings); FIX::SocketAcceptor acceptor (application, storeFactory, settings, logFactory /*optional*/); acceptor.start(); // while( condition == true ) { do something; } acceptor.stop(); return 0; } catch(FIX::ConfigError& e) { std::cout << e.what(); return 1; } }
Python
import quickfix if len(sys.argv) < 2: return fileName = sys.argv[1] try: settings = quickfix.SessionSettings(fileName) application = quickfix.MyApplication() storeFactory = quickfix.FileStoreFactory(settings) logFactory = quickfix.FileLogFactory(settings) acceptor = quickfix.SocketAcceptor(application, storeFactory, settings, logFactory) acceptor.start() # while condition == true: do something acceptor.stop() except quickfix.ConfigError, e: print e
Ruby
require 'quickfix_ruby' return if ARGV.length < 2 fileName = ARGV[0] begin settings = Quickfix::SessionSettings.new( fileName ) application = MyApplication.new storeFactory = Quickfix::FileStoreFactory.new( settings ) logFactory = Quickfix::ScreenLogFactory.new( settings ) acceptor = Quickfix::SocketAcceptor.new( application, storeFactory, settings, logFactory ) acceptor.start() # while condition == true: do something acceptor.stop() rescue Quickfix::ConfigError => e print e end
Configuration
A quickfix acceptor or initiator can maintain multiple FIX sessions. A FIX session is defined in QuickFIX as a unique combination of a BeginString (the FIX version number), a SenderCompID (your ID), and a TargetCompID (the ID of your counterparty). A SessionQualifier can also be use to disambiguate otherwise identical sessions.
The SessionSettings class has the ability to pull settings out of any c++ stream such as a file stream. You can also pass it a filename. If you decide to write your own components, (storage for a particular database, a new kind of connector etc...), you can also use this pass in settings.
A settings file is set up with two types of headings, a [DEFAULT] and a [SESSION] heading. [SESSION] tells QuickFIX that a new Session is being defined. [DEFAULT] is a where you can define settings that all sessions use by default. If you do not provide a setting that QuickFIX needs, it will throw a ConfigError telling you what setting is missing or improperly formatted.
Sample Settings File
# default settings for sessions [DEFAULT] ConnectionType=initiator ReconnectInterval=60 SenderCompID=TW # session definition [SESSION] # inherit ConnectionType, ReconnectInterval and SenderCompID from default BeginString=FIX.4.1 TargetCompID=ARCA StartTime=12:30:00 EndTime=23:30:00 HeartBtInt=20 SocketConnectPort=9823 SocketConnectHost=123.123.123.123 DataDictionary=somewhere/FIX41.xml [SESSION] BeginString=FIX.4.0 TargetCompID=ISLD StartTime=12:00:00 EndTime=23:00:00 HeartBtInt=30 SocketConnectPort=8323 SocketConnectHost=23.23.23.23 DataDictionary=somewhere/FIX40.xml [SESSION] BeginString=FIX.4.2 TargetCompID=INCA StartTime=12:30:00 EndTime=21:30:00 # overide default setting for RecconnectInterval ReconnectInterval=30 HeartBtInt=30 SocketConnectPort=6523 SocketConnectHost=3.3.3.3 # (optional) alternate connection ports and hosts to cycle through on failover SocketConnectPort1=8392 SocketConnectHost1=8.8.8.8 SocketConnectPort2=2932 SocketConnectHost2=12.12.12.12 DataDictionary=somewhere/FIX42.xml
QuickFIX Settings
ID | Description | Valid Values | Default |
---|---|---|---|
Session | |||
BeginString | Version of FIX this session should use | FIXT.1.1 FIX.4.4 FIX.4.3 FIX.4.2 FIX.4.1 FIX.4.0 |
|
SenderCompID | Your ID as associated with this FIX session | case-sensitive alpha-numeric string | |
TargetCompID | Counter parties ID as associated with this FIX session | case-sensitive alpha-numeric string | |
SessionQualifier | Additional qualifier to disambiguate otherwise identical sessions | case-sensitive alpha-numeric string | |
DefaultApplVerID | Required only for FIXT 1.1 (and newer). Ignored for earlier transport versions. Specifies the default application version ID for the session. This can either be the ApplVerID enum (see the ApplVerID field) or the BeginString for the default version. | FIX.5.0SP2 FIX.5.0SP1 FIX.5.0 FIX.4.4 FIX.4.3 FIX.4.2 FIX.4.1 FIX.4.0 9 8 7 6 5 4 3 2 |
|
ConnectionType | Defines if session will act as an acceptor or an initiator | initiator acceptor |
|
StartTime | Time of day that this FIX session becomes activated | time in the format of HH:MM:SS, time is represented in UTC | |
EndTime | Time of day that this FIX session becomes deactivated | time in the format of HH:MM:SS, time is represented in UTC | |
StartDay | For week long sessions, the starting day of week for the session. Use in combination with StartTime. | Day of week in English using any abbreviation (i.e. mo, mon, mond, monda, monday are all valid) | |
EndDay | For week long sessions, the ending day of week for the session. Use in combination with EndTime. | Day of week in English using any abbreviation (i.e. mo, mon, mond, monda, monday are all valid) | |
LogonTime | Time of day that this session logs on | time in the format of HH:MM:SS, time is represented in UTC | SessionTime value |
LogoutTime | Time of day that this session logs out | time in the format of HH:MM:SS, time is represented in UTC | EndTime value |
LogonDay | For week long sessions, the day of week the session logs on. Use in combination with LogonTime. | Day of week in English using any abbreviation (i.e. mo, mon, mond, monda, monday are all valid) | StartDay value |
LogoutDay | For week long sessions, the day of week the session logs out. Use in combination with LogoutTime. | Day of week in English using any abbreviation (i.e. mo, mon, mond, monda, monday are all valid) | EndDay value |
UseLocalTime | Indicates StartTime and EndTime are expressed in localtime instead of UTC. Times in messages will still be set to UTC as this is required by the FIX specifications. | Y N |
N |
MillisecondsInTimeStamp | Determines if milliseconds should be added to timestamps. Only available for FIX.4.2 and greater. | Y N |
Y |
TimestampPrecision | Used to set the fractional part of timestamp. Allowable values are 0 to 9. If set, overrrides MillisecondsInTimeStamp. | 0-9 | |
SendRedundantResendRequests | If set to Y, QuickFIX will send all necessary resend requests, even if they appear redundant. Some systems will not certify the engine unless it does this. When set to N, QuickFIX will attempt to minimize resend requests. This is particularly useful on high volume systems. | Y N |
N |
ResetOnLogon | Determines if sequence numbers should be reset when recieving a logon request. Acceptors only. | Y N |
N |
ResetOnLogout | Determines if sequence numbers should be reset to 1 after a normal logout termination. | Y N |
N |
ResetOnDisconnect | Determines if sequence numbers should be reset to 1 after an abnormal termination. | Y N |
N |
RefreshOnLogon | Determines if session state should be restored from persistence layer when logging on. Useful for creating hot failover sessions. | Y N |
N |
ID | Description | Valid Values | Default |
Validation | |||
UseDataDictionary | Tell session whether or not to expect a data dictionary. You should always use a DataDictionary if you are using repeating groups. | Y N |
Y |
DataDictionary | XML definition file for validating incoming FIX
messages. If no DataDictionary is supplied, only basic
message validation will be done This setting should only be used with FIX transport versions older than FIXT.1.1. See TransportDataDictionary and AppDataDictionary for FIXT.1.1 settings. |
valid XML data dictionary file, QuickFIX comes with
the following defaults in the spec directory FIX44.xml FIX43.xml FIX42.xml FIX41.xml FIX40.xml |
|
TransportDataDictionary | XML definition file for validating admin (transport)
messages. This setting is only valid for FIXT.1.1 (or
newer) sessions. See DataDictionary for older transport versions (FIX.4.0 - FIX.4.4) for additional information. |
valid XML data dictionary file, QuickFIX comes with
the following defaults in the spec directory FIXT1.1.xml |
|
AppDataDictionary |
XML definition file for validating application
messages. This setting is only valid for FIXT.1.1 (or
newer) sessions. See DataDictionary for older transport versions (FIX.4.0 - FIX.4.4) for additional information. This setting supports the possibility of a custom application data dictionary for each session. This setting would only be used with FIXT 1.1 and new transport protocols. This setting can be used as a prefix to specify multiple application dictionaries for the FIXT transport. For example: DefaultApplVerID=FIX.4.2 # For default application version ID AppDataDictionary=FIX42.xml # For nondefault application version ID # Use BeginString suffix for app version AppDataDictionary.FIX.4.4=FIX44.xml |
valid XML data dictionary file, QuickFIX comes with
the following defaults in the spec directory FIX50SP2.xml FIX50SP1.xml FIX50.xml FIX44.xml FIX43.xml FIX42.xml FIX41.xml FIX40.xml |
|
ValidateLengthAndChecksum | If set to N, messages with incorrect length or checksum fields will not be rejected. You can also use this to force acceptance of repeating groups without a data dictionary. In this scenario you will not be able to access all repeating groups. | Y N |
Y |
ValidateFieldsOutOfOrder | If set to N, fields that are out of order (i.e. body fields in the header, or header fields in the body) will not be rejected. Useful for connecting to systems which do not properly order fields. | Y N |
Y |
ValidateFieldsHaveValues | If set to N, fields without values (empty) will not be rejected. Useful for connecting to systems which improperly send empty tags. | Y N |
Y |
ValidateUserDefinedFields | If set to N, user defined fields will not be rejected if they are not defined in the data dictionary, or are present in messages they do not belong to. | Y N |
Y |
PreserveMessageFieldsOrder | Should the order of fields in the main outgoing message body be preserved or not (as defined in the configuration file). Default: only groups specified order is preserved. | Y N |
N |
CheckCompID | If set to Y, messages must be received from the counterparty with the correct SenderCompID and TargetCompID. Some systems will send you different CompIDs by design, so you must set this to N. | Y N |
Y |
CheckLatency | If set to Y, messages must be received from the counterparty within a defined number of seconds (see MaxLatency). It is useful to turn this off if a system uses localtime for it's timestamps instead of GMT. | Y N |
Y |
MaxLatency | If CheckLatency is set to Y, this defines the number of seconds latency allowed for a message to be processed. Default is 120. | positive integer | 120 |
Miscellaneous |
|||
ID | Description | Valid Values | Default |
HttpAcceptPort | Port to listen to HTTP requests. Pointing a browser to this port will bring up a control panel. Must be in DEFAULT section. | positive integer | |
Initiator |
|||
ID | Description | Valid Values | Default |
ReconnectInterval | Time between reconnection attempts in seconds. Only used for initiators | positive integer | 30 |
HeartBtInt | Heartbeat interval in seconds. Only used for initiators. | positive integer | |
LogonTimeout | Number of seconds to wait for a logon response before disconnecting. | positive integer | 10 |
LogoutTimeout | Number of seconds to wait for a logout response before disconnecting. | positive integer | 2 |
SocketConnectPort | Socket port for connecting to a session. Only used with a SocketInitiator | positive integer | |
SocketConnectHost | Host to connect to. Only used with a SocketInitiator | valid IP address in the format of x.x.x.x or a domain name | |
SocketConnectPort<n> | Alternate socket ports for connecting to a session for failover, where n is a positive integer. (i.e.) SocketConnectPort1, SocketConnectPort2... must be consecutive and have a matching SocketConnectHost[n] | positive integer | |
SocketConnectHost<n> | Alternate socket hosts for connecting to a session for failover, where n is a positive integer. (i.e.) SocketConnectHost1, SocketConnectHost2... must be consecutive and have a matching SocketConnectPort[n] | valid IP address in the format of x.x.x.x or a domain name | |
SocketNodelay | Indicates a socket should be created with TCP_NODELAY. Currently, this must be defined in the [DEFAULT] section. | Y N |
N |
SocketSendBufferSize | Indicates the size of SO_SNDBUF. Currently, this must be defined in the [DEFAULT] section. | positive integer | 0 |
SocketReceiveBufferSize | Indicates the size of SO_RCVBUF. Currently, this must be defined in the [DEFAULT] section. | positive integer | 0 |
ID | Description | Valid Values | Default |
Acceptor | |||
SocketAcceptPort | Socket port for listening to incoming connections, Only used with a SocketAcceptor | positive integer, valid open socket port. Currently, this must be defined in the [DEFAULT] section. | |
SocketReuseAddress | Indicates a socket should be created with SO_REUSADDR, Only used with a SocketAcceptor | Y N |
Y |
SocketNodelay | Indicates a socket should be created with TCP_NODELAY. Currently, this must be defined in the [DEFAULT] section. | Y N |
N |
ID | Description | Valid Values | Default |
Storage | |||
PersistMessages | If set to N, no messages will be persisted. This will force QuickFIX to always send GapFills instead of resending messages. Use this if you know you never want to resend a message. Useful for market data streams. | Y Y |
N |
File | |||
FileStorePath | Directory to store sequence number and message files. | valid directory for storing files, must have write access | |
MYSQL | |||
MySQLStoreDatabase | Name of MySQL database to access for storing messages and session state. | valid database for storing files, must have write access and correct DB shema | quickfix |
MySQLStoreUser | User name logging in to MySQL database. | valid user with read/write access to appropriate tables in database | root |
MySQLStorePassword | Users password. | correct MySQL password for user | empty password |
MySQLStoreHost | Address of MySQL database. | valid IP address in the format of x.x.x.x or a domain name | localhost |
MySQLStorePort | Port of MySQL database. | positive integer | standard MySQL port |
MySQLStoreUseConnectionPool | Use database connection pools. When possible, sessions will share a single database connection. Otherwise each session gets its own connection. | Y N |
N |
POSTGRESQL | |||
PostgreSQLStoreDatabase | Name of PostgreSQL database to access for storing messages and session state. | valid database for storing files, must have write access and correct DB shema | quickfix |
PostgreSQLStoreUser | User name logging in to PostgreSQL database. | valid user with read/write access to appropriate tables in database | postgres |
PostgreSQLStorePassword | Users password. | correct PostgreSQL password for user | empty password |
PostgreSQLStoreHost | Address of MySQL database. | valid IP address in the format of x.x.x.x or a domain name | localhost |
PostgreSQLStorePort | Port of PostgreSQL database. | positive integer | standard PostgreSQL port |
PostgreSQLStoreUseConnectionPool | Use database connection pools. When possible, sessions will share a single database connection. Otherwise each session gets its own connection. | Y N |
N |
ODBC | |||
OdbcStoreUser | User name logging in to ODBC database. | valid user with read/write access to appropriate tables in database. Ignored if UID is in the OdbcStoreConnectionString. | sa |
OdbcStorePassword | Users password. | correct ODBC password for user. Ignored if PWD is in the OdbcStoreConnectionString. | empty password |
OdbcStoreConnectionString | ODBC connection string for database | Valid ODBC connection string | DATABASE=quickfix;DRIVER={SQL Server};SERVER=(local); |
ID | Description | Valid Values | Default |
Logging | |||
File | |||
FileLogPath | Directory to store logs. | valid directory for storing files, must have write access | |
FileLogBackupPath | Directory to store backup logs. | valid directory for storing backup files, must have write access | |
Screen | |||
ScreenLogShowIncoming | Print incoming messages to standard out. | Y N |
Y |
ScreenLogShowOutgoing | Print outgoing messages to standard out. | Y N |
Y |
ScreenLogShowEvents | Print events to standard out. | Y N |
Y |
MYSQL | |||
MySQLLogDatabase | Name of MySQL database to access for logging. | valid database for storing files, must have write access and correct DB shema | quickfix |
MySQLLogUser | User name logging in to MySQL database. | valid user with read/write access to appropriate tables in database | root |
MySQLLogPassword | Users password. | correct MySQL password for user | empty password |
MySQLLogHost | Address of MySQL database. | valid IP address in the format of x.x.x.x or a domain name | localhost |
MySQLLogPort | Port of MySQL database. | positive integer | standard MySQL port |
MySQLLogUseConnectionPool | Use database connection pools. When possible, sessions will share a single database connection. Otherwise each session gets its own connection. | Y N |
N |
MySQLLogIncomingTable | Name of table where incoming messages will be logged. | Valid table with correct schema. | messages_log |
MySQLLogOutgoingTable | Name of table where outgoing messages will be logged. | Valid table with correct schema. | messages_log |
MySQLLogEventTable | Name of table where events will be logged. | Valid table with correct schema. | event_log |
POSTGRESQL | |||
PostgreSQLLogDatabase | Name of PostgreSQL database to access for logging. | valid database for storing files, must have write access and correct DB shema | quickfix |
PostgreSQLLogUser | User name logging in to PostgreSQL database. | valid user with read/write access to appropriate tables in database | postgres |
PostgreSQLLogPassword | Users password. | correct PostgreSQL password for user | empty password |
PostgreSQLLogHost | Address of PostgreSQL database. | valid IP address in the format of x.x.x.x or a domain name | localhost |
PostgreSQLLogPort | Port of PostgreSQL database. | positive integer | standard PostgreSQL port |
PostgreSQLLogUseConnectionPool | Use database connection pools. When possible, sessions will share a single database connection. Otherwise each session gets its own connection. | Y N |
N |
PostgresSQLLogIncomingTable | Name of table where incoming messages will be logged. | Valid table with correct schema. | messages_log |
PostgresSQLLogOutgoingTable | Name of table where outgoing messages will be logged. | Valid table with correct schema. | messages_log |
PostgresSQLLogEventTable | Name of table where events will be logged. | Valid table with correct schema. | event_log |
ODBC | |||
OdbcLogUser | User name logging in to ODBC database. | valid user with read/write access to appropriate tables in database | sa |
OdbcLogPassword | Users password. | correct ODBC password for user. Ignored if UID is in the OdbcLogConnectionString. | empty password |
OdbcLogConnectionString | ODBC connection string for database | Valid ODBC connection string. Ignored if PWD is in the OdbcStoreConnectionString. | DATABASE=quickfix;DRIVER={SQL Server};SERVER=(local); |
OdbcLogIncomingTable | Name of table where incoming messages will be logged. | Valid table with correct schema. | messages_log |
OdbcLogOutgoingTable | Name of table where outgoing messages will be logged. | Valid table with correct schema. | messages_log |
OdbcLogEventTable | Name of table where events will be logged. | Valid table with correct schema. | event_log |
ID | Description | Valid Values | Default |
SSL | |||
Parameters have to be defined in the DEFAULT section. | |||
SSLProtocol | This directive can be used to control the SSL protocol flavors the application
should use when establishing its environment. The available (case-insensitive) protocols are: SSLv2 This is the Secure Sockets Layer (SSL) protocol, version 2.0. It is the original SSL protocol as designed by Netscape Corporation. SSLv3 This is the Secure Sockets Layer (SSL) protocol, version 3.0. It is the successor to SSLv2 and the currently (as of February 1999) de-facto standardized SSL protocol from Netscape Corporation. It's supported by almost all popular browsers. TLSv1 This is the Transport Layer Security (TLS) protocol, version 1.0. TLSv1_1 This is the Transport Layer Security (TLS) protocol, version 1.1. TLSv1_2 This is the Transport Layer Security (TLS) protocol, version 1.2. all This is a shortcut for +SSLv2 +SSLv3 +TLSv1 +TLSv1_1 +TLSv1_2 and a convenient way for enabling all protocols except one when used in combination with the minus sign on a protocol as the example above shows. Example: enable all but not SSLv2 SSL_PROTOCOL = all -SSLv2 |
all -SSLv2 | |
SSLCipherSuite | This complex directive uses a colon-separated cipher-spec string consisting
of OpenSSL cipher specifications to configure the Cipher Suite the client is
permitted to negotiate in the SSL handshake phase. Notice that this directive
can be used both in per-server and per-directory context. In per-server
context it applies to the standard SSL handshake when a connection is
established. In per-directory context it forces a SSL renegotation with the
reconfigured Cipher Suite after the HTTP request was read but before the HTTP
response is sent. An SSL cipher specification in cipher-spec is composed of 4 major attributes plus a few extra minor ones. Key Exchange Algorithm: RSA or Diffie-Hellman variants. Authentication Algorithm: RSA, Diffie-Hellman, DSS or none. Cipher/Encryption Algorithm: DES, Triple-DES, RC4, RC2, IDEA or none. MAC Digest Algorithm: MD5, SHA or SHA1. For more details refer to mod_ssl documentation. Example: RC4+RSA:+HIGH: |
HIGH:!RC4 | |
CertificationAuthoritiesFile | This directive sets the all-in-one file where you can assemble the Certificates of Certification Authorities (CA) whose clients you deal with. | ||
CertificationAuthoritiesDirectory | This directive sets the directory where you keep the Certificates of Certification Authorities (CAs) whose clients you deal with. | ||
Acceptor | |||
ServerCertificateFile | This directive points to the PEM-encoded Certificate file and optionally also to the corresponding RSA or DSA Private Key file for it (contained in the same file). | ||
ServerCertificateKeyFile | This directive points to the PEM-encoded Private Key file. If the Private Key is not combined with the Certificate in the server certificate file, use this additional directive to point to the file with the stand-alone Private Key. | ||
CertificateVerifyLevel | This directive sets the Certificate verification level. It applies to the authentication process used in the standard SSL handshake when a connection is established. 0 implies do not verify. 1 implies verify. | ||
CertificateRevocationListFile | This directive sets the all-in-one file where you can assemble the Certificate Revocation Lists (CRL) of Certification Authorities (CA) whose clients you deal with. | ||
CertificateRevocationListDirectory | This directive sets the directory where you keep the Certificate Revocation Lists (CRL) of Certification Authorities (CAs) whose clients you deal with. | ||
Initiator | |||
ClientCertificateFile | This directive points to the PEM-encoded Certificate file and optionally also to the corresponding RSA or DSA Private Key file for it (contained in the same file). | ||
ClientCertificateKeyFile | This directive points to the PEM-encoded Private Key file. If the Private Key is not combined with the Certificate in the server certificate file, use this additional directive to point to the file with the stand-alone Private Key. |
Validation
QuickFIX will validate and reject any poorly formed messages before they can reach your application. An XML file defines what messages, fields, and values a session supports.
Several standard files are in included in the spec directory.
The skeleton of a definition file looks like this.
<fix type="FIX" major="4" minor="1"> <header> <field name="BeginString" required="Y"/> ... </header> <trailer> <field name="CheckSum" required="Y"/> ... </trailer> <messages> <message name="Heartbeat" msgtype="0" msgcat="admin"> <field name="TestReqID" required="N"/> </message> ... <message name="NewOrderSingle" msgtype="D" msgcat="app"> <field name="ClOrdID" required="Y"/> ... </message> ... </messages> <fields> <field number="1" name="Account" type="CHAR" /> ... <field number="4" name="AdvSide" type="CHAR"> <value enum="B" description="BUY" /> <value enum="S" description="SELL" /> <value enum="X" description="CROSS" /> <value enum="T" description="TRADE" /> </field> ... </fields> </fix>
The validator will not reject conditionally required fields because the rules for them are not clearly defined. Using a conditionally required field that is not preset will cause a message to be rejected.
Working With Messages
Receiving Messages
Most of the messages you will be interested in looking at will be arriving in your overloaded fromApp function of your application. All messages have a header and a trailer. If you want to see those fields, you must call getHeader() or getTrailer() on the message to access them.
Type Safe Messages And Fields
QuickFIX has a class for all messages defined in the standard spec.
C++
void fromApp( const FIX::Message& message, const FIX::SessionID& sessionID ) throw( FIX::FieldNotFound&, FIX::IncorrectDataFormat&, FIX::IncorrectTagValue&, FIX::UnsupportedMessageType& ) { crack(message, sessionID); } void onMessage( const FIX42::NewOrderSingle& message, const FIX::SessionID& ) { FIX::ClOrdID clOrdID; message.get(clOrdID); FIX::ClearingAccount clearingAccount; message.get(clearingAccount); } void onMessage( const FIX41::NewOrderSingle& message, const FIX::SessionID& ) { FIX::ClOrdID clOrdID; message.get(clOrdID); // compile time error!! field not defined in FIX41 FIX::ClearingAccount clearingAccount; message.get(clearingAccount); } void onMessage( const FIX42::OrderCancelRequest& message, const FIX::SessionID& ) { FIX::ClOrdID clOrdID; message.get(clOrdID); // compile time error!! field not defined for OrderCancelRequest FIX::Price price; message.get(price); }
In order to use this you must use the MessageCracker
as a mixin to your application. This will provide you with the
crack function and allow you to overload specific
message functions. Any function you do not overload will by
default throw an UnsupportedMessageType exception
Define your application like this:
C++
#include "quickfix/Application.h" #include "quickfix/MessageCracker.h" class MyApplication : public FIX::Application, public FIX::MessageCracker
Type Safe Fields Only
Use the getField method to get any field from any message.
C++
void fromApp( const FIX::Message& message, const FIX::SessionID& sessionID ) throw( FIX::FieldNotFound&, FIX::IncorrectDataFormat&, FIX::IncorrectTagValue&, FIX::UnsupportedMessageType& ) { // retrieve value into field class FIX::Price price; message.getField(price); // another field... FIX::ClOrdID clOrdID; message.getField(clOrdID); }
Python
import quickfix def fromApp(self, message, sessionID): price = quickfix.Price() message.getField(price) clOrdID = quickfix.ClOrdID() message.getField(clOrdID)
Ruby
require 'quickfix_ruby' def fromApp(message, sessionID): price = Quickfix::Price.new() message.getField(price) clOrdID = Quickfix::ClOrdID.new() message.getField(clOrdID) end
No Type Safety
Create your own fields using tag numbers.
C++
void fromApp( const FIX::Message& message, const FIX::SessionID& sessionID ) throw( FIX::FieldNotFound&, FIX::IncorrectDataFormat&, FIX::IncorrectTagValue&, FIX::UnsupportedMessageType& ) { // retreive value into string with integer field ID std::string value; value = message.getField(44); // retrieve value into a field base with integer field ID FIX::FieldBase field(44, ""); message.getField(field); // retreive value with an enumeration, a little better message.getField(FIX::FIELD::Price); }
Python
import quickfix def fromApp(self, message, sessionID): field = quickfix.StringField(44) message.getField(field)
Ruby
require 'quickfix_ruby' def fromApp(message, sessionID): field = Quickfix::StringField.new(44) message.getField(field) end
Sending Messages
Messages can be sent to a counter party with the static Session::sendToTarget methods.
C++
// send a message that already contains a BeginString, SenderCompID, and a TargetCompID static bool sendToTarget( Message&, const std::string& qualifier = "" ) throw(SessionNotFound&); // send a message based on the sessionID, convenient for use // in fromApp since it provides a session ID for incoming // messages static bool sendToTarget( Message&, const SessionID& ) throw(SessionNotFound&); // append a SenderCompID and TargetCompID before sending static bool sendToTarget( Message&, const SenderCompID&, const TargetCompID&, const std::string& qualifier = "" ) throw(SessionNotFound&); // pass SenderCompID and TargetCompID in as strings static bool sendToTarget( Message&, const std::string&, const std::string&, const std::string& qualifier = "" ) throw(SessionNotFound&);
Python
def sendToTarget(message): def sendToTarget(message, qualifier): def sendToTarget(message, sessionID): def sendToTarget(message, senderCompID, targetCompID): def sendToTarget(message, senderCompID, targetCompID, qualifier):
Ruby
def sendToTarget(message) def sendToTarget(message, qualifier) def sendToTarget(message, sessionID) def sendToTarget(message, senderCompID, targetCompID) def sendToTarget(message, senderCompID, targetCompID, qualifier)
Type Safe Messages And Fields
Message constructors take in all required fields and adds the correct MsgType and BeginString for you. With the set method, the compiler will not let you add a field that is not a part of a message as defined by the spec.
C++
void sendOrderCancelRequest() { FIX41::OrderCancelRequest message( FIX::OrigClOrdID("123"), FIX::ClOrdID("321"), FIX::Symbol("LNUX"), FIX::Side(FIX::Side_BUY)); message.set(FIX::Text("Cancel My Order!")); FIX::Session::sendToTarget(message, SenderCompID("TW"), TargetCompID("TARGET")); }
Type Safe Field Only
The setField method allows you to add any field to any message.
C++
void sendOrderCancelRequest() { FIX::Message message; FIX::Header header& = message.getHeader(); header.setField(FIX::BeginString("FIX.4.2")); header.setField(FIX::SenderCompID(TW)); header.setField(FIX::TargetCompID("TARGET")); header.setField(FIX::MsgType(FIX::MsgType_OrderCancelRequest)); message.setField(FIX::OrigClOrdID("123")); message.setField(FIX::ClOrdID("321")); message.setField(FIX::Symbol("LNUX")); message.setField(FIX::Side(FIX::Side_BUY)); message.setField(FIX::Text("Cancel My Order!")); FIX::Session::sendToTarget(message); }
Python
from quickfix import * def sendOrderCancelRequest: message = quickfix.Message(); header = message.getHeader(); header.setField(quickfix.BeginString("FIX.4.2")) header.setField(quickfix.SenderCompID(TW)) header.setField(quickfix.TargetCompID("TARGET")) header.setField(quickfix.MsgType("D")) message.setField(quickfix.OrigClOrdID("123")) message.setField(quickfix.ClOrdID("321")) message.setField(quickfix.Symbol("LNUX")) message.setField(quickfix.Side(Side_BUY)) message.setField(quickfix.Text("Cancel My Order!")) Session.sendToTarget(message)
Ruby
require 'quickfix_ruby' def sendOrderCancelRequest message = Quickfix::Message.new(); header = message.getHeader(); header.setField(Quickfix::BeginString.new("FIX.4.2")) header.setField(Quickfix::SenderCompID.new(TW)) header.setField(Quickfix::TargetCompID.new("TARGET")) header.setField(Quickfix::MsgType.new("D")) message.setField(Quickfix::OrigClOrdID.new("123")) message.setField(Quickfix::ClOrdID.new("321")) message.setField(Quickfix::Symbol.new("LNUX")) message.setField(Quickfix::Side.new(Side.BUY)) message.setField(Quickfix::Text.new("Cancel My Order!")) Quickfix::Session.sendToTarget(message) end
No Type Safety
You can also use setField to pass in primitives.
C++
void sendOrderCancelRequest() { FIX::Message message; // BeginString message.getHeader().setField(8, "FIX.4.2"); // SenderCompID message.getHeader().setField(49, "TW"); // TargetCompID, with enumeration message.getHeader().setField(FIX::FIELD::TargetCompID, "TARGET"); // MsgType message.getHeader().setField(35, 'F'); // OrigClOrdID message.setField(41, "123"); // ClOrdID message.setField(11, "321"); // Symbol message.setField(55, "LNUX"); // Side, with value enumeration message.setField(54, FIX::Side_BUY); // Text message.setField(58, "Cancel My Order!"); FIX::Session::sendToTarget(message); }
Python
from quickfix import * def sendOrderCancelRequest: message = quickfix.Message() # BeginString message.getHeader().setField(quickfix.StringField(8, "FIX.4.2")) # SenderCompID message.getHeader().setField(quickfix.StringField(49, "TW")) # TargetCompID, with enumeration message.getHeader().setField(quickfix.StringField(56, "TARGET")) # MsgType message.getHeader().setField(quickfix.CharField(35, 'F')) # OrigClOrdID message.setField(quickfix.StringField(41, "123")) # ClOrdID message.setField(quickfix.StringField(11, "321")) # Symbol message.setField(quickfix.StringField(55, "LNUX")) # Side, with value enumeration message.setField(quickfix.CharField(54, quickfix.Side_BUY)) # Text message.setField(quickfix.StringField(58, "Cancel My Order!")) Session.sendToTarget(message);
Ruby
require 'quickfix_ruby' def sendOrderCancelRequest message = Quickfix::Message.new() # BeginString message.getHeader().setField(Quickfix::StringField.new(8, "FIX.4.2")) # SenderCompID message.getHeader().setField(Quickfix::StringField.new(49, "TW")) # TargetCompID, with enumeration message.getHeader().setField(Quickfix::StringField.new(56, "TARGET")) # MsgType message.getHeader().setField(Quickfix::CharField.new(35, 'F')) # OrigClOrdID message.setField(Quickfix::StringField.new(41, "123")) # ClOrdID message.setField(Quickfix::StringField.new(11, "321")) # Symbol message.setField(Quickfix::StringField.new(55, "LNUX")) # Side, with value enumeration message.setField(Quickfix::CharField.new(54, quickfix.Side_BUY)) # Text message.setField(Quickfix::StringField.new(58, "Cancel My Order!")) Quickfix::Session.sendToTarget(message) end
Repeating Groups
Creating Messages With Repeating Groups
When a message is created the required field declaring the number of repeating groups is set to zero. QuickFIX will automatically increment field for you as you add groups.
C++
// create a market data message FIX42::MarketDataSnapshotFullRefresh message(FIX::Symbol("QF")); // repeating group in the form of MessageName::NoField FIX42::MarketDataSnapshotFullRefresh::NoMDEntries group; group.set(FIX::MDEntryType('0')); group.set(FIX::MDEntryPx(12.32)); group.set(FIX::MDEntrySize(100)); group.set(FIX::OrderID("ORDERID")); message.addGroup(group); // no need to create a new group class if we are reusing the fields group.set(FIX::MDEntryType('1')); group.set(FIX::MDEntryPx(12.32)); group.set(FIX::MDEntrySize(100)); group.set(FIX::OrderID("ORDERID")); message.addGroup(group);
Python
import quickfix import quickfix42 message = quickfix42.MarketDataSnapshotFullRefresh() group = quickfix42.MarketDataSnapshotFullRefresh().NoMDEntries() group.setField(quickfix.MDEntryType('0')) group.setField(quickfix.MDEntryPx(12.32)) group.setField(quickfix.MDEntrySize(100)) group.setField(quickfix.OrderID("ORDERID")) message.addGroup( group ) group.setField(quickfix.MDEntryType('1')) group.setField(quickfix.MDEntryPx(12.32)) group.setField(quickfix.MDEntrySize(100)) group.setField(quickfix.OrderID("ORDERID")) message.addGroup( group )
Ruby
require 'quickfix_ruby' require 'quickfix42' message = Quickfix42::MarketDataSnapshotFullRefresh.new() group = Quickfix42::MarketDataSnapshotFullRefresh::NoMDEntries.new(); group.setField(Quickfix::MDEntryType.new('0')) group.setField(Quickfix::MDEntryPx.new(12.32)) group.setField(Quickfix::MDEntrySize.new(100)) group.setField(Quickfix::OrderID.new("ORDERID")) message.addGroup( group ) group.setField(Quickfix::MDEntryType.new('1')) group.setField(Quickfix::MDEntryPx.new(12.32)) group.setField(Quickfix::MDEntrySize.new(100)) group.setField(Quickfix::OrderID.new("ORDERID")) message.addGroup( group )
Reading Messages With Repeating Groups
To pull a group out of a message you need to supply the group object you wish to pull out. You should first inspect the number of entries field (which the group is named after) to get the total number of groups. The message that was created above is now parsed in this example.
C++
// should be 2 FIX::NoMDEntries noMDEntries; message.get(noMDEntries); FIX42::MarketDataSnapshotFullRefresh::NoMDEntries group; FIX::MDEntryType MDEntryType; FIX::MDEntryPx MDEntryPx; FIX::MDEntrySize MDEntrySize; FIX::OrderID orderID; message.getGroup(1, group); group.get(MDEntryType); group.get(MDEntryPx); group.get(MDEntrySize); group.get(orderID); message.getGroup(2, group); group.get(MDEntryType); group.get(MDEntryPx); group.get(MDEntrySize); group.get(orderID);
Python
import quickfix import quickfix42 noMDEntries = quickfix.NoMDEntries() message.getField(noMDEntries) group = quickfix42.MarketDataSnapshotFullRefresh.NoMDEntries() MDEntryType = quickfix.MDEntryType() MDEntryPx = quickfix.MDEntryPx() MDEntrySize = quickfix.MDEntrySize() orderID = quickfix.OrderID(); message.getGroup(1, group); group.getField(MDEntryType); group.getField(MDEntryPx); group.getField(MDEntrySize); group.getField(orderID); message.getGroup(2, group); group.getField(MDEntryType); group.getField(MDEntryPx); group.getField(MDEntrySize); group.getField(orderID);
Ruby
require 'quickfix_ruby' require 'quickfix42' noMDEntries = Quickfix::NoMDEntries.new() message.getField(noMDEntries) group = Quickfix42::MarketDataSnapshotFullRefresh.NoMDEntries.new() MDEntryType = Quickfix::MDEntryType.new() MDEntryPx = Quickfix::MDEntryPx.new() MDEntrySize = Quickfix::MDEntrySize.new() orderID = Quickfix::OrderID.new(); message.getGroup(1, group); group.getField(MDEntryType); group.getField(MDEntryPx); group.getField(MDEntrySize); group.getField(orderID); message.getGroup(2, group); group.getField(MDEntryType); group.getField(MDEntryPx); group.getField(MDEntrySize); group.getField(orderID);
User Defined Fields
FIX allows users to define fields not defined in the specifications. How can QuickFIX be used to set and get user defined fields? Well one answer would be to use the non-type safe set and get fields like so:
C++
message.setField(6123, "value"); message.getField(6123);
Python
message.setField(quickfix.StringField(6123, "value")) field = message.getField(quickfix.StringField(6123)
Ruby
message.setField(Quickfix::StringField.new(6123, "value")) field = message.getField(Quickfix::StringField(6123).new())
QuickFIX also provides supplies macros for creating typesafe field objects.
C++
#include "quickfix/Field.h" namespace FIX { USER_DEFINE_STRING(MyStringField, 6123); USER_DEFINE_PRICE(MyPriceField, 8756); }
Python
from quickfix import * class MyStringField(quickfix.StringField): def __init__(self, data = None) if data == None: quickfix.StringField.__init__(self, 6123) else: quickfix.StringField.__init__(self, 6123, data) class MyPriceField(quickfix.DoubleField): def __init__(self, data = None) if data == None: quickfix.DoubleField.__init__(self, 8756) else: quickfix.DoubleField.__init__(self, 8756, data)
Ruby
require 'quickfix_ruby' class MyStringField < Quickfix::StringField def initialize( data = nil ) if( data == nil ) super(6123) else super(6123, data) end end end class MyDoubleField < Quickfix::DoubleField def initialize( data = nil ) if( data == nil ) super(8756) else super(8756, data) end end end
User defined fields must be declared within the FIX namespace. Now, elsewhere in your application, you can write code like this:
C++
MyStringField stringField("string"); MyPriceField priceField(14.54); message.setField(stringField); message.setField(priceField); message.getField(stringField); message.getField(priceField);
Ruby
stringField = MyStringField("string") priceField = MyPriceField(14.54) message.setField(stringField) message.setField(priceField) message.getField(stringField) message.getField(priceField)
Python
stringField = MyStringField.new("string") priceField = MyPriceField.new(14.54) message.setField(stringField) message.setField(priceField) message.getField(stringField) message.getField(priceField)
These macros allow you to define fields of all supported FIX types. Keep in mind you can write fields of any type types as long as you supply a new macro and convertor that can convert your type to and from a string.
C++
USER_DEFINE_STRING( NAME, NUM ) USER_DEFINE_CHAR( NAME, NUM ) USER_DEFINE_PRICE( NAME, NUM ) USER_DEFINE_INT( NAME, NUM ) USER_DEFINE_AMT( NAME, NUM ) USER_DEFINE_QTY( NAME, NUM ) USER_DEFINE_CURRENCY( NAME, NUM ) USER_DEFINE_MULTIPLEVALUESTRING( NAME, NUM ) USER_DEFINE_EXCHANGE( NAME, NUM ) USER_DEFINE_UTCTIMESTAMP( NAME, NUM ) USER_DEFINE_BOOLEAN( NAME, NUM ) USER_DEFINE_LOCALMKTDATE( NAME, NUM ) USER_DEFINE_DATA( NAME, NUM ) USER_DEFINE_FLOAT( NAME, NUM ) USER_DEFINE_PRICEOFFSET( NAME, NUM ) USER_DEFINE_MONTHYEAR( NAME, NUM ) USER_DEFINE_DAYOFMONTH( NAME, NUM ) USER_DEFINE_UTCDATE( NAME, NUM ) USER_DEFINE_UTCTIMEONLY( NAME, NUM ) USER_DEFINE_NUMINGROUP( NAME, NUM ) USER_DEFINE_SEQNUM( NAME, NUM ) USER_DEFINE_LENGTH( NAME, NUM ) USER_DEFINE_PERCENTAGE( NAME, NUM ) USER_DEFINE_COUNTRY( NAME, NUM )
Example Applications
QuickFIX comes with several example applications in the quickfix/examples directory.
ordermatch is a c++ server that will match and execute limit orders
tradeclient is a little c++ console based trading client.
banzai is a Java GUI based trading client.
executor is a server that fills every limit order it receives.Executor has been implemented in C++, Python and Ruby.
Testing
Unit Tests
QuickFIX comes with a comprehensive suite of automated unit tests. These tests are run by a framework called UnitTest++. UnitTest++ allows developers to test C++ code by writing code that calls functions on objects and asserting correct behavior. These test verifies not only that the code works correctly, but also that it works the same on all platforms.
This sample shows the setup and execution of the test that verifies the Parser object can correctly extract messages from a stream.
struct readFixMessageFixture { readFixMessageFixture() { fixMsg1 = "8=FIX.4.2\0019=12\00135=A\001108=30\00110=31\001"; fixMsg2 = "8=FIX.4.2\0019=17\00135=4\00136=88\001123=Y\00110=34\001"; fixMsg3 = "8=FIX.4.2\0019=19\00135=A\001108=30\0019710=8\00110=31\001"; object.addToStream( fixMsg1 + fixMsg2 + fixMsg3 ); } std::string fixMsg1; std::string fixMsg2; std::string fixMsg3; Parser object; }; TEST_FIXTURE(readFixMessageFixture, readFixMessage) { std::string readFixMsg1; CHECK( object.readFixMessage( readFixMsg1 ) ); CHECK_EQUAL( fixMsg1, readFixMsg1 ); std::string readFixMsg2; CHECK( object.readFixMessage( readFixMsg2 ) ); CHECK_EQUAL( fixMsg2, readFixMsg2 ); std::string readFixMsg3; CHECK( object.readFixMessage( readFixMsg3 ) ); CHECK_EQUAL( fixMsg3, readFixMsg3 ); }
Acceptance Tests
QuickFIX also has a scriptable test runner that comes with a series of automated acceptance test. The basic tests that come with QuickFIX are based off of the FIX Session-level Test Cases and Expected Behaviors document produced by the FIX protocol organization. These tests verify that QuickFIX adheres to the FIX specifications. The automated nature of these tests guarantees that future releases of QuickFIX will not break any current functionality.
Perhaps even more importantly is how these test are used to drive the development of QuickFIX. Before a line of code is written in support of a protocol feature, one of these tests is written. This test first approach sets up a goal for developers who will have objective verification that they correctly implemented the standard.
Below is an example of a test script that tests the engines behavior when it receives a NewSeqNo value that is less than the expected MsgSeqNum.
iCONNECT I8=FIX.4.235=A34=149=TW52=>TIME>56=ISLD98=0108=30 E8=FIX.4.29=5735=A34=149=ISLD52=00000000-00:00:0056=TW98=0108=3010=0 # sequence reset without gap fill flag (default to N) I8=FIX.4.235=434=049=TW52=>TIME>56=ISLD36=1 E8=FIX.4.29=11235=334=249=ISLD52=00000000-00:00:0056=TW45=058=Value is incorrect (out of range) for this tag372=4373=510=0 I8=FIX.4.235=134=249=TW52=>TIME>56=ISLD112=HELLO E8=FIX.4.29=5535=034=349=ISLD52=00000000-00:00:0056=TW112=HELLO10=0 # sequence reset without gap fill flag (default to N) I8=FIX.4.235=434=049=TW52=>TIME>56=ISLD36=1123=N E8=FIX.4.29=11235=334=449=ISLD52=00000000-00:00:0056=TW45=058=Value is incorrect (out of range) for this tag372=4373=510=0 I8=FIX.4.235=134=349=TW52=>TIME>56=ISLD112=HELLO E8=FIX.4.29=5535=034=549=ISLD52=00000000-00:00:0056=TW112=HELLO10=0 iDISCONNECT
In these script there are two types of commands, action commands and messages commands. Action commands begin with lowercase letters while message command begin with uppercase letters.
Action Commands
i<ACTION> - initiates an actione<ACTION> - expect (wait for) an action
Supported actions are:
iCONNECT - initiate connection to a FIX acceptor
eCONNECT - expect a connection from a FIX initiator
iDISCONNECT - initiate a disconnect
eDISCONNECT - expect a disconnect
Message Commands
I<MESSAGE> - initiate (send) a messageE<MESSAGE> - expect (wait for) a message
When using the I command, you do not need to add the Length(9) or the CheckSum(10) fields, they will be added for you with the correct values in the appropriate locations. The only time you would add these fields is if you intentionally wish to make them incorrect.
The I command also provides a TIME macro for fields. By setting a field equal to <TIME>, the current system time will be placed in the field. (i.e. 52=>TIME>). You can also use offsets such as 52=<TIME-120> or 52=<TIME+15> in order to set the time plus or minus some seconds from the current time.
The E command verifies that you have received the correct message. This command will compare the values of each field to make sure they are correct. Some fields cannot be verified deterministically before run-time such as the SendingTime and CheckSum fields. These fields can be added to the fields.fmt file where a regular expression can be defined to at least verify the field is in the correct format. For example:
10=\d{3}
, checksum must be exactly three digits
52=\d{8}-\d{2}:\d{2}:\d{2}
, sending time must be in the form of
DDDDDDDD-DD:DD:DD
where D is a digit.
Either command can contain a FILE macro which puts the contents of a file into a field (i.e. 58=<FILE:test.txt>)